Mongo vs. Postgres – Real-Life Comparison – Part 2
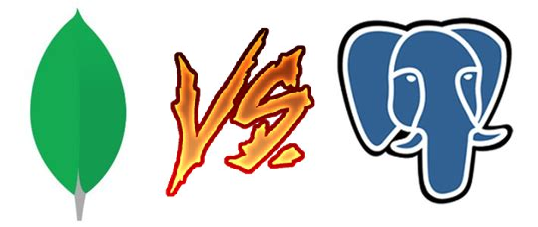
In case you haven’t read the first installment, I recommend checking it out before diving into the topics here. In this continuation, I’ll discuss a range of subjects that often come up when comparing MongoDB and PostgreSQL. The second part will discuss:
- Extensions
- Indexing
- Backup & Recovery
- Practical Max Size per Node
- Sharding
- Monitoring
- Connection Pooling
- Proxies
- Dev/Admin Tools
Let’s dive in!
Extensions
CopyEdit
One of the key advantages of PostgreSQL is how easily it can be extended. Postgres supports hundreds—if not thousands—of community-driven extensions that add or modify functionality without requiring you to modify the core database code. To use an extension, you can usually install a Linux package like postgres-contrib (often preinstalled by default), then enable the extension with a simple command:
psql -d your_database -c "CREATE EXTENSION pgcrypto";
Here, pgcrypto
is just one example that adds encryption functions. There are countless other extensions for JSON handling, specialized indexing, or even entire sharding solutions like Citus, which effectively transforms Postgres into a horizontally scalable database. It’s worth noting that not every Postgres extension ships in the core “contrib” bundle; many are maintained externally, often on GitHub or specialized repositories.
By contrast, MongoDB doesn’t offer a comparable mechanism for third-party extensions. If you hear about Percona’s Mongo distribution, that isn’t really an extension—it’s a separate fork of MongoDB, similar to how some Postgres forks exist (like Greenplum or Vertica). In other words, Postgres outperforms Mongo in flexibility for adding new features over time.
Indexing
Databases are only as powerful as their ability to retrieve data quickly, and both Mongo and Postgres provide robust indexing mechanisms. Mongo’s built-in indexes—like single-field, compound, multikey, geospatial, text, unique, partial, and sparse—cover most common scenarios. There’s also a TTL (Time to Live) index type, though its primary function is to automatically remove documents, not necessarily to speed up queries.
PostgreSQL supports its own set of index types (B-tree, Hash, GiST, SP-GiST, GIN, BRIN), which might sound smaller in comparison, but Postgres is extensible here, too. You can add specialized index types—such as Bloom indexes or Trigram indexes—for niche use cases. PostgreSQL also has a sophisticated query planner that can leverage these various indexes intelligently, especially if you feed it the right statistics.
In a typical JSON scenario, suppose you have this Mongo document:
{"toy_name": "dog", "can_do": ["eat", "sleep", "bark"]}
If you want to find which toys can “bark,” you might create a Mongo index on the can_do
field, allowing a query like:
lua
db.toys.find({"can_do": "bark"});
In Postgres, you can store that JSON in a column (e.g., json_column_name
) and then create a GIN index:
CREATE INDEX toys_can_do
ON toys
USING GIN ((json_column_name->'can_do') jsonb_path_ops);
Both databases handle such queries efficiently. Yet Postgres’s support for extensions provides more choices if you need unusual or advanced indexing strategies, while Mongo’s index options remain fairly comprehensive for typical document-based workloads.
Backup and Recovery
A quick note: dumping and restoring data (with pg_dump
/pg_restore
in Postgres or mongodump
/mongorestore
in Mongo) isn’t usually considered a robust, point-in-time backup strategy. Those tools are best for smaller environments or quick migrations.
Real backups in both databases generally happen at the file/block level. In Mongo, you place the database into a backup mode that essentially locks out writes to ensure consistency, then back up the files on disk. With Postgres, you do something similar—often referred to as “backup mode” or “pg_start_backup
” in older versions—yet Postgres allows the database to remain writable during this procedure. Mongo typically enforces a stricter lock, making Postgres more flexible for production environments that can’t afford downtime or read-only periods.
Incremental backups are another big deal. Postgres has a concept of WAL (Write-Ahead Log), enabling continuous archiving and point-in-time recovery out of the box. Mongo’s community edition lacks built-in incremental backups, so you either need the paid MongoDB Enterprise version or a third-party tool for advanced restore scenarios.
Sharded environments amplify these differences. With Postgres solutions like Citus, you can still do consistent, centralized backups across the cluster. With Mongo, you often have to orchestrate separate backups of each shard (each one being a separate replica set), then hope you can line them up to the same consistent timestamp. While it’s possible, it can get complicated.
Practical Max Size per Node
A frequent question is how large a single node can grow before performance degrades or management becomes unwieldy. From a purely technical standpoint, both Mongo and Postgres can handle huge datasets, limited mainly by the file system or the hardware itself. However, real-world considerations often encourage setting an upper size to avoid burdensome maintenance or slow responses.
In many Postgres deployments, around 5 TB per node is a comfortable ceiling before DBAs consider sharding. For Mongo, some practitioners advise keeping it closer to 1 TB per node. This isn’t a hard rule—it’s more about how each database handles concurrency and resources. Mongo runs primarily on a single mongod
process, while Postgres can spread work among multiple processes and allocate a dedicated process per connection. Also, Postgres has table partitioning, which allows you to “drop” older partitions quickly, whereas Mongo’s TTL or capped collections delete data in the background, which might be less efficient for very large data rollouts.
Sharding
Sharding is the horizontal partitioning of data across multiple nodes to scale out. Both Mongo and Postgres do it, but in different ways. Postgres has solutions like Postgres-XL, Greenplum, or Citus to spread data across multiple machines. These maintain ACID properties and full SQL compliance. You can also do a simpler “poor man’s sharding” with Foreign Data Wrappers, albeit with fewer automatic features.
Mongo’s built-in sharding relies on multiple replica sets, each acting as a shard. A minimal production sharded cluster might need at least 10 nodes: two replica sets (each with three data nodes, so 6 total), a config server replica set (3 more nodes), plus a mongos
router process. This can be more involved to maintain compared to a compact Citus or FDW-based Postgres cluster, which might only need 4 nodes. Mongo’s balancer, which distributes data among shards, also has a reputation for requiring careful tweaking of partition keys.
For many scenarios, Postgres’s sharding (especially with Citus) feels easier to manage, keeps high availability in mind, and still lets you use SQL for queries. Mongo’s sharding can also be powerful, but the overhead of managing multiple replica sets and a config server is something to consider.
Monitoring
Both databases offer the ability to track performance and resource usage. Postgres has a wealth of metadata views, such as pg_stat_activity
, along with extensive third-party monitoring options. Popular tools like pgAdmin, Grafana (with the Postgres plugin), or specialized solutions can easily ingest these stats.
Mongo also has monitoring commands and built-in statistics, but the ecosystem of third-party monitoring tools is somewhat smaller compared to Postgres. It’s not that Mongo lacks the data to be monitored—it’s more that the community and commercial landscape for Postgres is broader, offering countless specialized solutions.
Connection Pooling
Databases can be overwhelmed by too many connections. A pooling layer ensures that idle connections don’t hog resources and that active ones are served efficiently. Postgres traditionally relies on external tools like pgBouncer or pgpool, which you can set up once and apply to all apps connecting to the database. This approach requires no custom driver logic—just install and configure the pooling service on your server.
Mongo, by contrast, expects you to handle connection pooling mostly in the driver layer, such as in Spring Boot or Python’s Mongo libraries. Some developers prefer this, as it keeps everything in the application’s ecosystem. Others, however, appreciate the simpler, “set-it-and-forget-it” method of a dedicated pooling process. If you lean toward the second camp, Postgres has you covered.
Proxies
Postgres often relies on external proxies (like HAProxy or GoBetween) for routing connections to the primary in a high-availability cluster. These proxies can also route or load-balance based on custom rules, though that requires some expertise in configuration. Mongo achieves a similar effect via URI connection parameters, with read/write concerns defining how queries are distributed among replicas. Some people find Mongo’s built-in approach simpler, while others like the granular control of a standalone proxy. It ultimately depends on whether you prefer “batteries included” or a more customizable setup.
Development and Administration Tools
Both databases have grown robust ecosystems. Postgres is recognized for its wealth of administration tools—pgAdmin, DBeaver, DataGrip, and numerous others. Mongo has also seen improvements in its tooling, though the variety remains smaller. Tools like MongoDB Compass offer a decent graphical interface, and there are third-party solutions as well, but the Postgres ecosystem is still considered broader.
What this means in practice is that for certain complex tasks—especially around migrations, advanced index planning, or performance tuning—Postgres users may find more specialized or mature utilities at their disposal. Mongo’s ecosystem is catching up, but if you’re used to the enormous range of Postgres tools, you might find Mongo’s selection comparatively limited.
Final Thoughts
That wraps up Part 2 of this extended Mongo vs. Postgres comparison. We covered a lot: how each database handles extensions and indexing, different approaches to backup and recovery, scaling your node size, sharding architectures, monitoring strategies, connection pooling, proxies, and the overall tool ecosystem.
My own take is that Postgres often wins for long-term flexibility, deeper extension support, simpler backup methods, and a more unified approach to big data or sharding. Mongo stands out for highly fluid document structures, powerful JSON-oriented updates, and a built-in sharding method that can handle immense data volumes once it’s properly set up (albeit with more overhead).
I hope this helps you decide which database suits your needs better or at least gives you a clearer idea of where each one excels. In my next article, I plan to explore a slightly different topic: comparing the cost-effectiveness of cloud-based databases (like Amazon RDS) versus on-premises Postgres. Stay tuned—I’m looking forward to seeing which option might surprise us all.
Similar posts
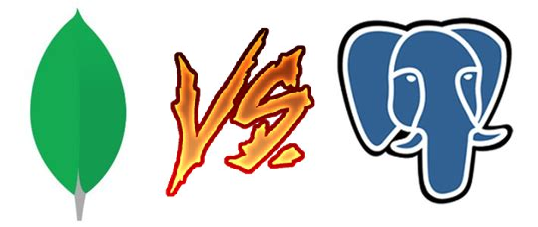
Mongo vs. Postgres – Real-Life Comparison – Part 1
Are you asking which database is better—or which is better for you?
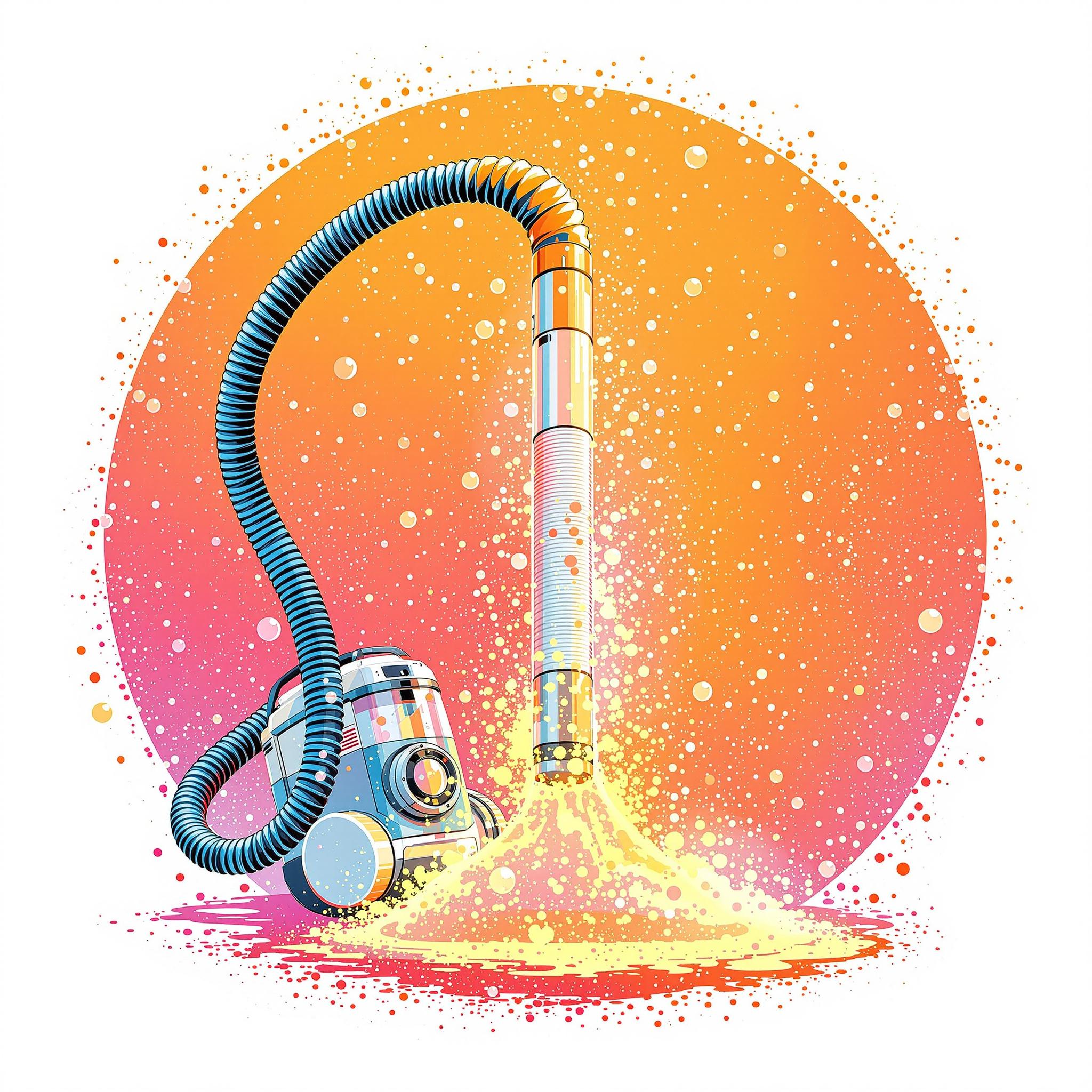
Misconfigured PostgreSQL Vacuum
Find out why VACUUM is more than just cleaning—it's a critical part of your database's well-being.
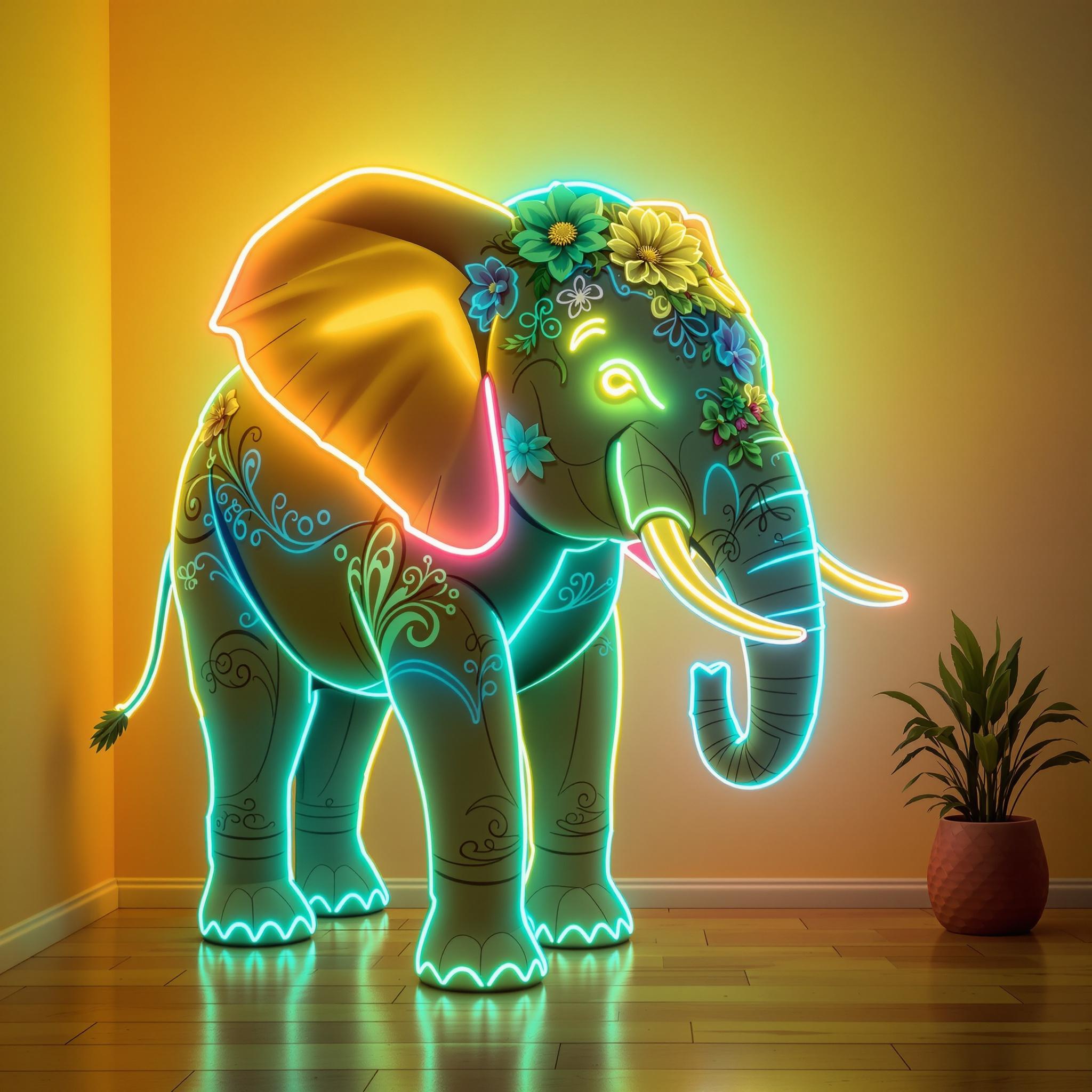
Can't ignore the Elephant in the room
Don’t ignore the elephant in the room—especially when it’s PostgreSQL.